项目创建
这个项目以提取minist数据集转换成图片为例,逐步指导创建python项目的方法。
1、创建一个虚拟环境
pip install virtualenv
python -m virtualenv minist
或
virtualenv mnist #需要根据安装后的提示设置环境变量
minist\Scripts\activate
2、安装程序需要依赖的库
pip install --trusted-host mirrors.cloud.tencent.com tensorflow tensorflow_datasets Pillow
3、使用vscode打开项目文件夹并创建源代码文件夹
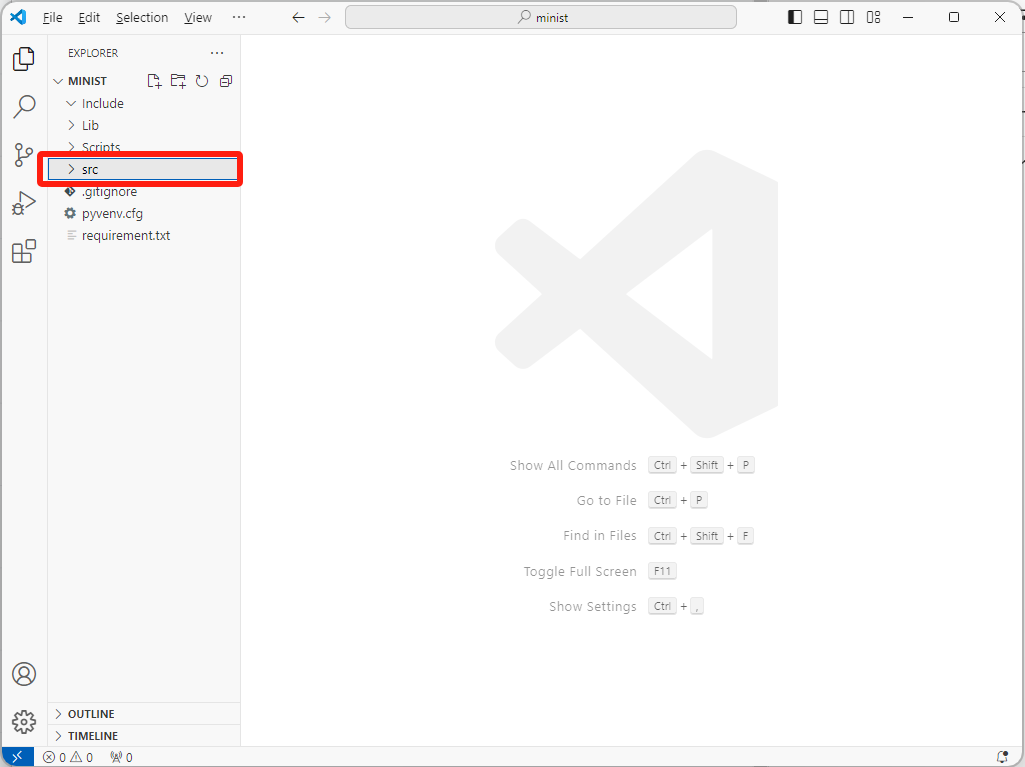
4、编写代码(minst_save_imag.py)
# encoding: utf-8
# -*- coding: utf8 -*-
import tensorflow as tf
import tensorflow_datasets as tfds
import tqdm as tqdm
from PIL import Image
import numpy as np
with open('../MINIST_data/train-labels.idx1-ubyte','rb') as file_labels:
# 读取文件判断minist文件的魔数是否为2049,以判断加载的文件是否为合法的minist的标签文件
magic_number = int.from_bytes(file_labels.read(4),'big')
if magic_number != 2049:
raise ValueError('Invalid MINIST label files')
#读取接下来的四个字节,获取图像标签数量
num_label_images = int.from_bytes(file_labels.read(4),'big')
print(num_label_images)
images_labels = []
with tqdm.tqdm(total=num_label_images,desc='查找图片标签') as pbar:
for _ in range(num_label_images):
images_label = int.from_bytes(file_labels.read(1),'big')
images_labels.append(images_label)
pbar.update(1)
with open('../MINIST_data/train-images.idx3-ubyte','rb') as file_trains:
# 获取MINIST镜像文件的魔数,判断是否为合法的镜像文件
magic_number = int.from_bytes(file_trains.read(4),'big')
if magic_number != 2051:
raise ValueError('Invalid MINIST image file')
# 获取存放的图像数量
num_images = int.from_bytes(file_trains.read(4),'big'); #60000
# 获取每个图像的像素行数
num_rows = int.from_bytes(file_trains.read(4),'big') #28
# 获取每个图像的像素列数
num_cols = int.from_bytes(file_trains.read(4),'big') #28
images = []
with tqdm.tqdm(total=num_images,desc="整理图片数据") as pbar:
# 提取图片
for _ in range(num_images):
image = []
# 提取图像的所有像素值
for _ in range(num_rows * num_cols):
pixel = int.from_bytes(file_trains.read(1),'big')
image.append(pixel)
images.append(np.array(image).reshape(num_rows,num_cols))
pbar.update(1)
# 将上述整理出的信息转存为图片
with tqdm.tqdm(total=len(images),desc='下载图片数据') as pbar:
for index,image in enumerate(images):
image_to_save = Image.fromarray(image).convert('L')
file_name = str(images_labels[index]) + "_" + str(index)
image_to_save.save('../MINIST_data/images/' + file_name + ".png")
pbar.update(1)
5、运行代码
cd D:\programming\python\minist\src
python minst_save_imag.py
6、生成requirement.txt,方便管理依赖
pip freeze > requirement.txt
下载源代码后,如果首次运行,则需要执行命令,安装必要的依赖
pip install --trusted-host mirrors.cloud.tencent.com -r requirement.txt
错误解决
1、在vscode下执行激活虚拟环境时,产生如下错误:
D:\programming\python\minist\Scripts\activate.ps1,因为在此系统上禁止运行脚本…。
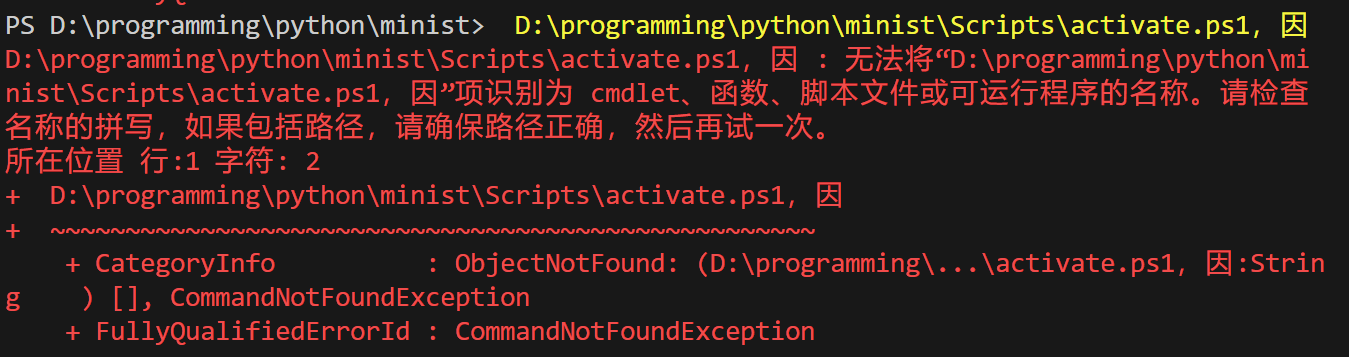
解决:
以管理身份运行PowerShell,并且输入命令:Set-ExecutionPolicy RemoteSigned
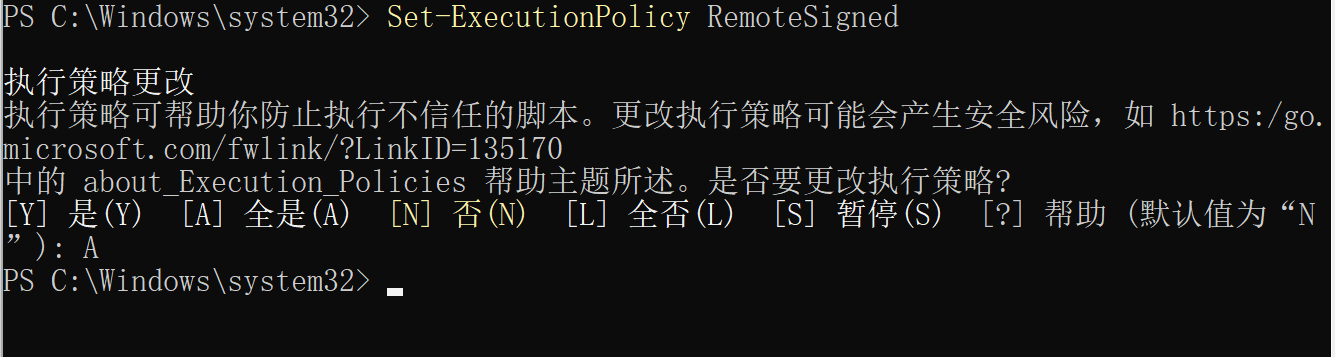
2、Defaulting to user installation because normal site-packages is not writeable

Defaulting to user installation because normal site-packages is not writeable
Requirement already satisfied: virtualenv in c:\users\98350\appdata\local\packages\pythonsoftwarefoundation.python.3.12_qbz5n2kfra8p0\localcache\local-packages\python312\site-packages (20.26.3)
解决:
将文件夹【site-packages】的只读变为非只读状态。
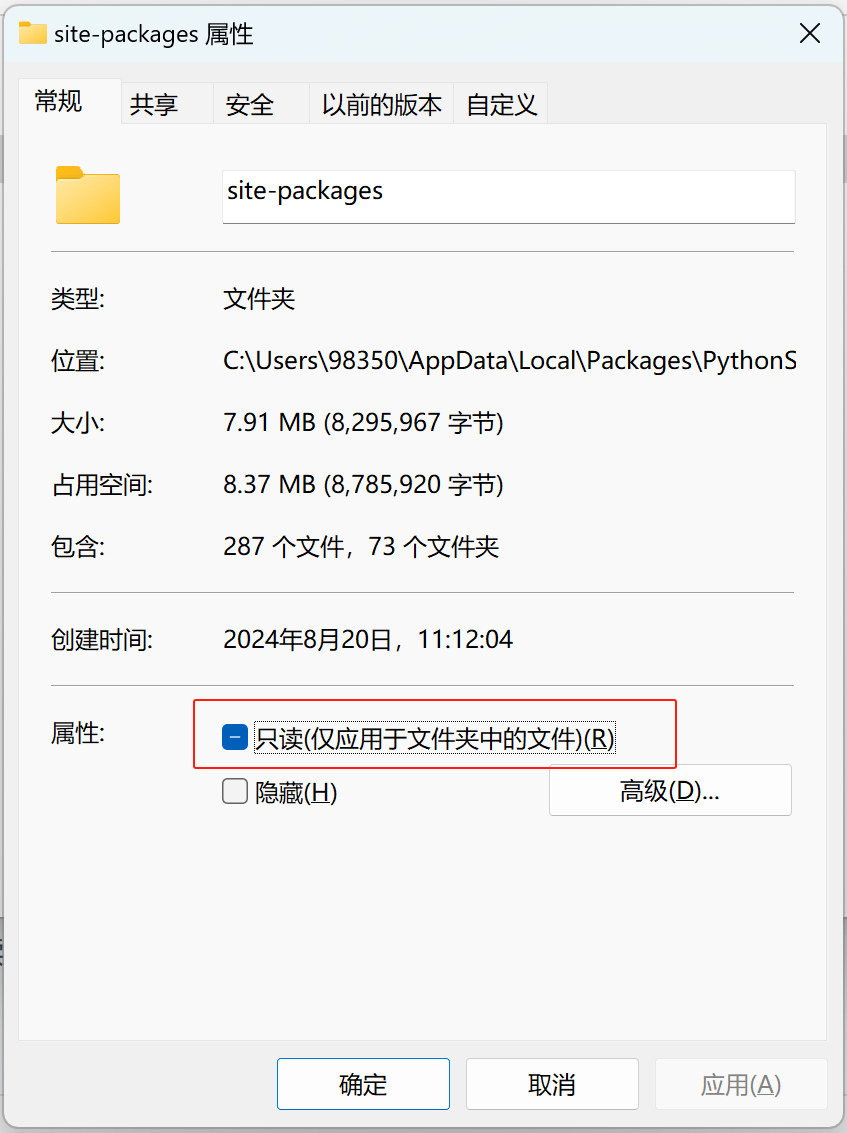