父模块
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>2022.0.4</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-alibaba-dependencies</artifactId>
<version>2022.0.0.0</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.0.9</version>
</parent>
子模块—mobile服务
引入依赖
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-bootstrap</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-config</artifactId>
<version>2022.0.0.0</version>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<!-- <dependency>-->
<!-- <groupId>org.springframework.boot</groupId>-->
<!-- <artifactId>spring-boot-starter-actuator</artifactId>-->
<!-- </dependency>-->
<!-- <dependency>-->
<!-- <groupId>org.springframework.cloud</groupId>-->
<!-- <artifactId>spring-cloud-commons</artifactId>-->
<!-- </dependency>-->
</dependencies>
bootstrap.yaml
spring:
profiles:
active: dev
bootstrap-dev.yaml
spring:
application:
name: mobile
cloud:
nacos:
config:
server-addr: 192.168.58.2:8848
namespace: htgw
group: dev
file-extension: yaml
nacos配置中心的配置
server:
port: 8002
servlet:
context-path: /mobile
session:
timeout: 900
spring:
application:
name: mobile
webflux:
base-path: /mobile
cloud:
nacos:
discovery:
server-addr: 192.168.58.2:8848
namespace: htgw
启动类
package com.dokbok.htgw.gateway.htgw.mobile;
import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.bootstrap.BootstrapConfiguration;
import org.springframework.context.ApplicationContext;
@BootstrapConfiguration
@SpringBootApplication
@Slf4j
public class MobileApplication {
public static void main(String[] args) {
ApplicationContext ctx = SpringApplication.run(MobileApplication.class, args);
String[] activeProfiles = ctx.getEnvironment().getActiveProfiles();
for (String profile : activeProfiles) {
log.warn("Spring Boot 使用profile为:{}" , profile);
}
}
}
对外服务的Controller类:
package com.dokbok.htgw.gateway.htgw.mobile.test;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.HashMap;
import java.util.Map;
@RestController
@RequestMapping("/test")
public class TestController {
@RequestMapping("hello")
public Object hello(){
Map<String,Object> map = new HashMap<>();
map.put("tdj","乔");
return map;
}
}
查看nacos的注册的服务列表
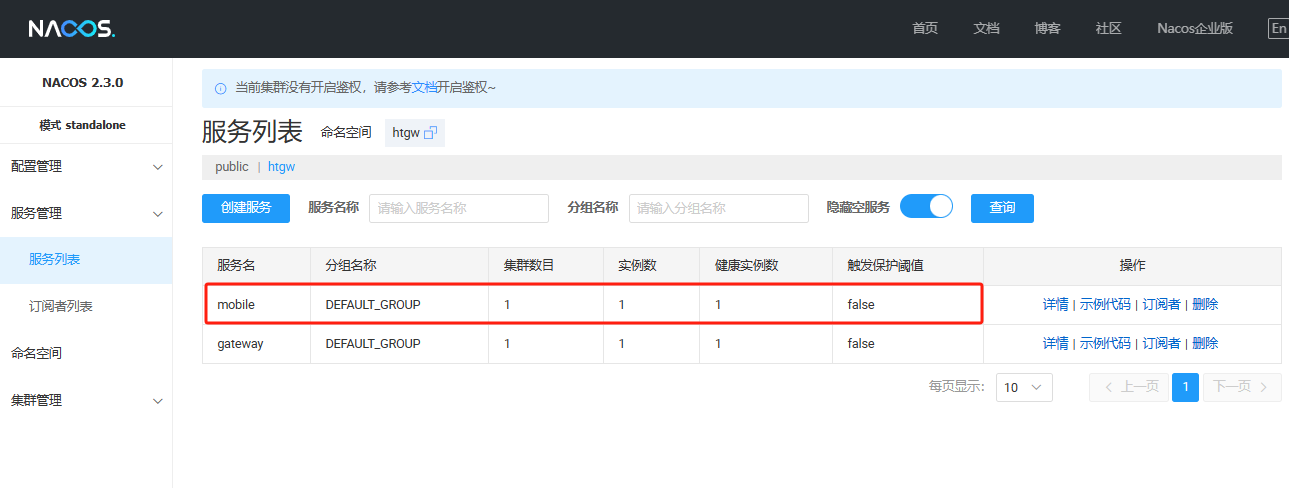
网关服务
引入依赖
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-bootstrap</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-loadbalancer</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-config</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
</dependencies>
bootstrap.yaml
spring:
profiles:
active: dev
bootstrap-dev.yaml
spring:
application:
name: gateway
cloud:
nacos:
config:
server-addr: 192.168.58.2:8848
namespace: htgw
group: dev
file-extension: yaml
nacos配置中心的配置
server:
port: 8001
spring:
cloud:
nacos:
discovery:
server-addr: 192.168.58.2:8848
namespace: htgw
gateway:
routes:
- id: mobile
uri: lb://mobile
predicates:
- Path=/mobile/**
启动类:
package com.dokbok.htgw.gateway;
import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.bootstrap.BootstrapConfiguration;
import org.springframework.context.ApplicationContext;
@BootstrapConfiguration
@SpringBootApplication
@Slf4j
public class GatewayApplication {
public static void main(String[] args) {
ApplicationContext ctx = SpringApplication.run(GatewayApplication.class, args);
String[] activeProfiles = ctx.getEnvironment().getActiveProfiles();
for (String profile : activeProfiles) {
log.warn("Spring Boot 使用profile为:{}" , profile);
}
}
}
测试
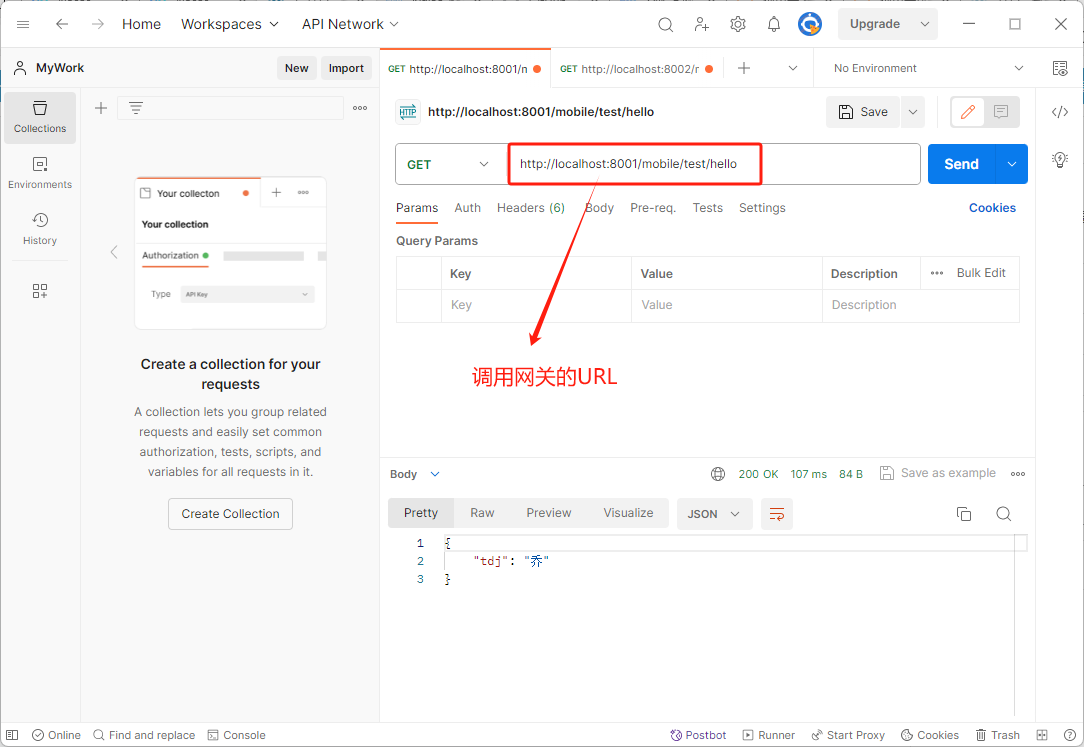