概述
本文章是SpringBoot上传文件的单元测试代码实现。一定要注意一些问题,要使用@RunWith(SpringRunner.class)进行注解,否则会导致Controller调用Service的时候出现空指针(Service没用被springboot初始化)。
要使用@SpringBootTest(classes = MinioApplication.class)来标识系统的主启动类,否则在运行单元测试的时候,会由于启动类没有启动而无法完成测试。
代码实现
1、引入对应的库
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-junit-jupiter</artifactId>
<scope>test</scope>
</dependency>
2、编写代码
import com.dokbok.minio.bucket.controller.FileController;
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.InjectMocks;
import org.mockito.Spy;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.http.MediaType;
import org.springframework.mock.web.MockMultipartFile;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.MvcResult;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
import org.springframework.test.web.servlet.result.MockMvcResultMatchers;
import org.springframework.test.web.servlet.setup.MockMvcBuilders;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
@RunWith(SpringRunner.class)
@SpringBootTest(classes = MinioApplication.class)
@AutoConfigureMockMvc
public class FileUploadTest {
private MockMvc mockMvc;
@Autowired
private FileController fileController;
@Before
public void init(){
mockMvc = MockMvcBuilders.standaloneSetup(fileController).build();
}
@Test
public void testFileUpload() throws FileNotFoundException {
try{
String fileName = "Oracle-coding-style.pdf";
File file = new File("D:\\development\\test\\" + fileName);
InputStream inputStream = new FileInputStream(file);
MockMultipartFile multipartFile = new MockMultipartFile(
"file",fileName,"multipart/form-data",inputStream);
MvcResult result = mockMvc.perform(
MockMvcRequestBuilders
.multipart("/file/upload")
.file(multipartFile)
.param("bucket","test")
.param("destPath","myfiles")
.contentType(MediaType.MULTIPART_FORM_DATA))
.andExpect(MockMvcResultMatchers.status().is(200)).andReturn();
Assert.assertEquals(200,result.getResponse().getStatus());
Assert.assertNotNull(result.getResponse().getContentAsString());
}catch (Exception e){
e.printStackTrace();
}
}
}
注:
- 需要测试的Controller一定要使用注入(@Autowired)的方式引入,不允许使用new关键字,否则会导致Controller内部调用的Service空指针
- param用于同步传入其他form-data参数
使用postman测试时的情况,用于与单元测试的代码进行对比:
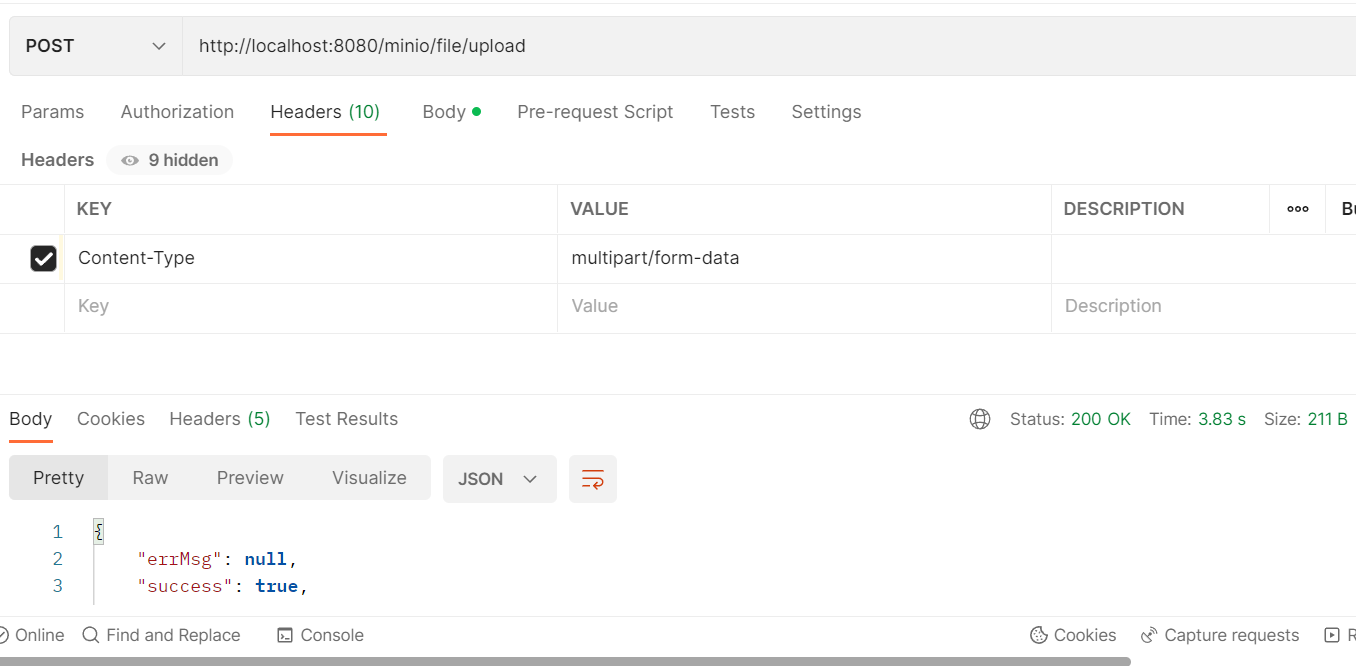
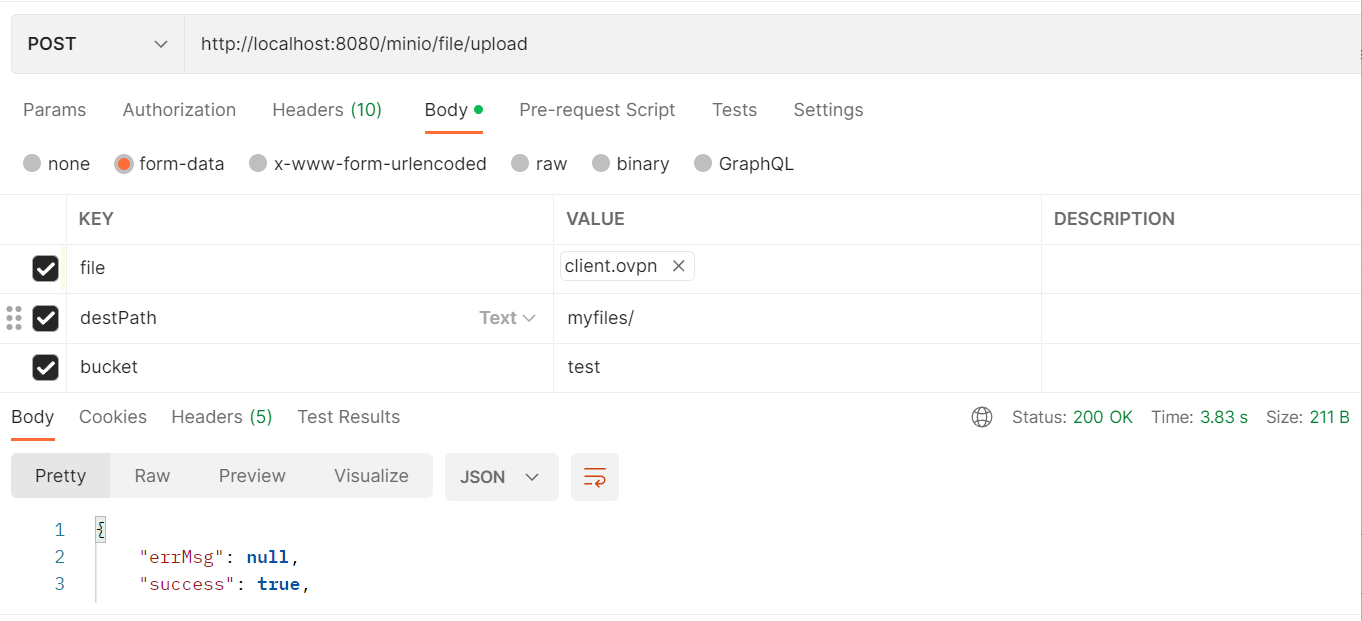